Building a Game Engine: A Step-by-Step Guide
===============================================
Creating a game engine from scratch can be a daunting task for many developers. However, it can also be an incredibly rewarding experience that allows you to learn more about the inner workings of games and how they are created. In this step-by-step guide, we will walk you through the process of building a basic game engine, from setting up your development environment to creating a simple game.
Table of Contents
-----------------
1. [Introduction](#introduction)
2. [Setting Up Your Development Environment](#development-environment)
3. [Understanding the Game Loop](#game-loop)
4. [Creating a Window and Rendering Graphics](#window-and-graphics)
5. [Input and Event Handling](#input-and-event)
6. [Creating a Simple Game](#simple-game)
Advertisement
7. [Conclusion](#conclusion)
Introduction
------------
A game engine is a software framework that provides developers with the tools and libraries necessary to create a game. It handles many of the low-level details such as rendering graphics, processing input, and managing game states. Building your own game engine can be a great way to learn more about game development and gain a deeper understanding of how games work.
Setting Up Your Development Environment
----------------------------------------
Before you can start building your game engine, you will need to set up your development environment. This includes choosing a programming language, an operating system, and any additional tools or libraries that you will need.
Programming Language
The first thing you will need to decide is which programming language you will use to build your game engine. Some popular choices include C++, C#, and Python. Each language has its own strengths and weaknesses, so choose one that you are comfortable with and that fits your needs.
Operating System
You will also need to decide which operating system you will be developing your game engine on. Most game engines are developed on Windows, but it is possible to create a game engine that runs on multiple operating systems.
Development Tools
There are many tools available that can help you with game development. Some of the most popular include:
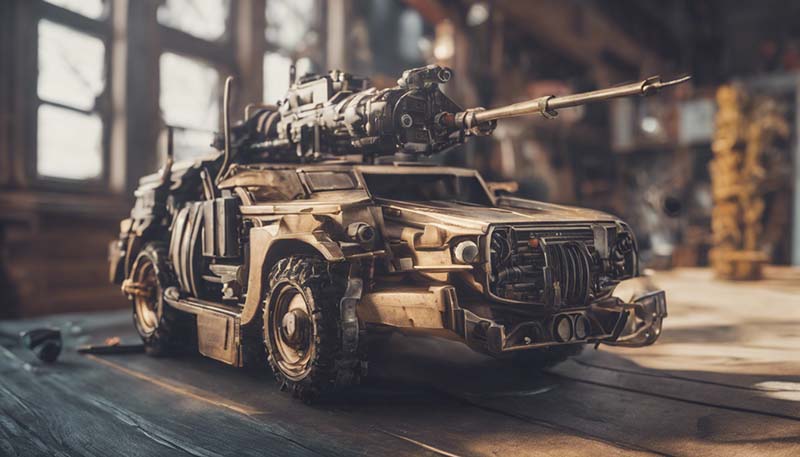
* **Visual Studio**: A powerful IDE that supports many programming languages and has many features that can help with game development.
* **Unity**: A popular game engine that provides a visual interface for creating games. It also has a large community and many resources available.
* **Unreal Engine**: Another popular game engine that is known for its high-quality graphics and support for a wide range of platforms.
Additional Libraries
Depending on the features you want to include in your game engine, you may need to use additional libraries. Some common libraries used in game development include:
* **OpenGL**: A library for rendering 3D graphics.
* **SDL**: A library for handling input and creating windows.
* **Box2D**: A physics engine for simulating realistic physics in games.
Understanding the Game Loop
---------------------------
The game loop is the core of any game engine. It is a continuous loop that runs as long as the game is running. The game loop is responsible for updating the game state, processing input, and rendering graphics.
Here is a basic outline of what the game loop looks like:
c
while (!gameOver) {
// Process input
// Update game state
// Render graphics
}
Processing Input
The first step in the game loop is to process input from the user. This can include keyboard and mouse input, as well as input from a game controller.
Updating Game State
After processing input, the game state is updated based on the input and any other factors such as physics or AI. This is where most of the logic for the game takes place.
Rendering Graphics
Finally, the game loop renders the graphics to the screen. This can include drawing 2D sprites, 3D models, and text.
Creating a Window and Rendering Graphics
-----------------------------------------
Once you have a basic understanding of the game loop, the next step is to create a window and start rendering graphics.
Creating a Window
Creating a window is a necessary step for any game engine. The window is where the game will be displayed to the user. There are many libraries available that can help you create a window, such as SDL or GLFW.
Here is an example of creating a window using SDL:
c
#include
int main(int argc, char* argv[]) {
SDL_Init(SDL_INIT_VIDEO);
SDL_Window* window = SDL_CreateWindow(\"Game Engine\", SDL_WINDOWPOS_CENTERED, SDL_WINDOWPOS_CENTERED, 800, 600, SDL_WINDOW_SHOWN);
SDL_Renderer* renderer = SDL_CreateRenderer(window, -1, SDL_RENDERER_ACCELERATED);
// Main game loop
bool gameOver = false;
while (!gameOver) {
SDL_Event event;
while (SDL_PollEvent(&event)) {
if (event.type == SDL_QUIT) {
gameOver = true;
}
}
// Clear the screen
SDL_SetRenderDrawColor(renderer, 0, 0, 0, 255);
SDL_RenderClear(renderer);
// Render graphics here
SDL_RenderPresent(renderer);
}
// Clean up
SDL_DestroyRenderer(renderer);
SDL_DestroyWindow(window);
SDL_Quit();
return 0;
}
Rendering Graphics
After creating a window, you can start rendering graphics. This can include drawing simple shapes like rectangles and circles, as well as more complex shapes like 3D models.
Here is an example of rendering a simple rectangle using SDL:
c
// Set the color of the rectangle
SDL_SetRenderDrawColor(renderer, 255, 0, 0, 255);
// Draw a rectangle
SDL_Rect rect = {100, 100, 200, 100};
SDL_RenderFillRect(renderer, &rect);
You can also use libraries like OpenGL to render more complex graphics like 3D models and textures.
Input and Event Handling
------------------------
Input and event handling is a crucial part of any game engine. It allows the game to respond to user input and other events such as collisions or timers.
Keyboard and Mouse Input
Most games require some form of keyboard and mouse input. There are many libraries available that can help you handle keyboard and mouse input, such as SDL or GLFW.
Here is an example of handling keyboard input using SDL:
c
while (!gameOver) {
SDL_Event event;
while (SDL_PollEvent(&event)) {
if (event.type == SDL_QUIT) {
gameOver = true;
} else if (event.type == SDL_KEYDOWN) {
switch (event.key.keysym.sym) {
case SDLK_UP:
// Handle up arrow key press
break;
case SDLK_DOWN:
// Handle down arrow key press
break;
// Add more cases for other keys as needed
}
}
}
// Update game state and render graphics
}
Game Controller Input
In addition to keyboard and mouse input, you may also want to support game controller input. This can be useful for games that are designed to be played with a controller.
Handling game controller input can be more complex than handling keyboard and mouse input, as there are many different types of controllers with different button layouts. However, libraries like SDL can help simplify the process.
Creating a Simple Game
----------------------
Now that you have a basic understanding of the game loop, creating a window, rendering graphics, and handling input, you can start creating a simple game.
Game Design
The first step in creating a game is to come up with a design. This can include deciding on the game\'s theme, mechanics, and overall structure.
Game State Management
One important aspect of game design is managing the game\'s state. This can include things like the current level, the player\'s health, and any other variables that are important to the game.
You can use a simple state machine to manage the game\'s state. For example:
c
enum GameState {
MainMenu,
Playing,
Paused,
GameOver
};
GameState currentState = MainMenu;
while (!gameOver) {
switch (currentState) {
case MainMenu:
// Render main menu and handle user input
break;
case Playing:
// Update game state and render graphics
break;
case Paused:
// Render pause menu and handle user input
break;
case GameOver:
// Render game over screen and handle user input
break;
}
}
Implementing Game Mechanics
Once you have a basic design and state management in place, you can start implementing the game\'s mechanics. This can include things like movement, physics, and collision detection.
Here is a simple example of implementing player movement:
c
struct Player {
float x, y;
float speed;
};
Player player;
player.x = 400;
player.y = 300;
player.speed = 500.0f;
while (!gameOver) {
while (SDL_PollEvent(&event)) {
if (event.type == SDL_KEYDOWN) {
switch (event.key.keysym.sym) {
case SDLK_LEFT:
player.x -= player.speed * deltaTime;
break;
case SDLK_RIGHT:
player.x += player.speed * deltaTime;
break;
// Add more cases for other keys as needed
}
}
}
// Update other game state and render graphics
// Clear the screen
SDL_SetRenderDrawColor(renderer, 0, 0, 0, 255);
SDL_RenderClear(renderer);
// Draw player
SDL_Rect playerRect = {(int)player.x, (int)player.y, 50, 50};
SDL_SetRenderDrawColor(renderer, 255, 0, 0, 255);
SDL_RenderFillRect(renderer, &playerRect);
SDL_RenderPresent(renderer);
}
Adding Graphics and Sound
Once the basic mechanics are in place, you can start adding graphics and sound to your game. This can include things like sprites, animations, and background music.
Here is an example of loading and rendering a sprite using SDL:
c
SDL_Texture* sprite;
sprite = SDL_CreateTextureFromImage(\"sprite.png\");
while (!gameOver) {
// Update game state and handle input
// Clear the screen
SDL_SetRenderDrawColor(renderer, 0, 0, 0, 255);
SDL_RenderClear(renderer);
// Draw sprite
SDL_Rect spriteRect = {player.x, player.y, 50, 50};
SDL_RenderCopy(renderer, sprite, NULL, &spriteRect);
SDL_RenderPresent(renderer);
}
Conclusion
----------
Building a game engine from scratch can be a challenging but rewarding experience. In this guide, we have covered the basics of setting up your development environment, understanding the game loop, creating a window and rendering graphics, handling input and events, and creating a simple game.
With these foundational concepts in place, you can start building more complex games and adding additional features to your game engine. Remember to keep learning and experimenting, as game development is a constantly evolving field with new techniques and technologies emerging all the time.
Good luck, and happy game development!